装饰器模式允许用户在不改变其结构的情况下向现有对象添加新功能。 这种类型的设计模式属于结构模式,因为这种模式充当现有类的包装器。
这种模式创建了一个装饰器类,它包装了原始类并提供了额外的功能,使类方法签名保持不变。
我们通过以下示例演示装饰器模式的使用,在该示例中,我们将使用某种颜色装饰形状而不改变形状类。
举例说明
我们将创建一个 Shape 接口和实现 Shape 接口的具体类。 然后我们将创建一个抽象装饰器类 ShapeDecorator 实现 Shape 接口并将 Shape 对象作为其实例变量。
RedShapeDecorator 是实现 ShapeDecorator 的具体类。
DecoratorPatternDemo,我们的演示类将使用 RedShapeDecorator 来装饰 Shape 对象。
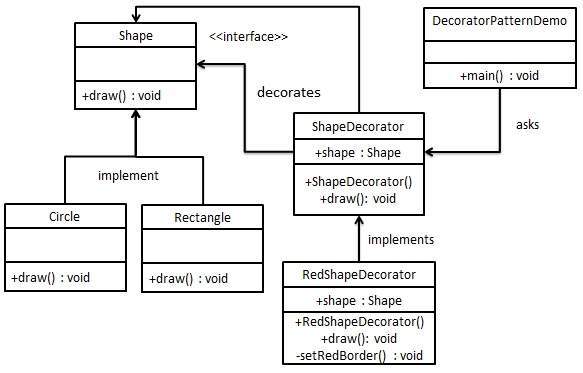
第一步
创建接口
public interface Shape {
void draw();
}
第二步
创建实现类
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Shape: Rectangle");
}
}
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Shape: Circle");
}
}
第三步
创建一个实现了相同接口的装饰虚类
public abstract class ShapeDecorator implements Shape {
protected Shape decoratedShape;
public ShapeDecorator(Shape decoratedShape){
this.decoratedShape = decoratedShape;
}
public void draw(){
decoratedShape.draw();
}
}
第四步
创建一个实现类
public class RedShapeDecorator extends ShapeDecorator {
public RedShapeDecorator(Shape decoratedShape) {
super(decoratedShape);
}
@Override
public void draw() {
decoratedShape.draw();
setRedBorder(decoratedShape);
}
private void setRedBorder(Shape decoratedShape){
System.out.println("Border Color: Red");
}
}
第五步
开始模拟实现装饰效果
public class DecoratorPatternDemo {
public static void main(String[] args) {
Shape circle = new Circle();
Shape redCircle = new RedShapeDecorator(new Circle());
Shape redRectangle = new RedShapeDecorator(new Rectangle());
System.out.println("Circle with normal border");
circle.draw();
System.out.println("\nCircle of red border");
redCircle.draw();
System.out.println("\nRectangle of red border");
redRectangle.draw();
}
}